Outerra forum
- July 22, 2025, 04:14:21 am
- Welcome, Guest
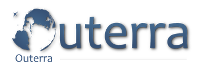
News:
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.