Outerra forum
- February 18, 2025, 05:23:00 pm
- Welcome, Guest
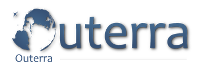
News:
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.