Outerra forum
- April 17, 2025, 03:08:41 pm
- Welcome, Guest
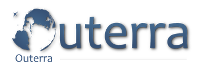
News:
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.
- Outerra forum >
- Outerra Engine >
- Development screen shots and videos >
- Importer and vehicle physics video
Author
Topic: Importer and vehicle physics video (Read 81138 times)
- Outerra forum >
- Outerra Engine >
- Development screen shots and videos >
- Importer and vehicle physics video