Outerra forum
- July 22, 2025, 10:38:24 am
- Welcome, Guest
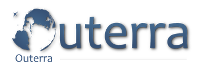
News:
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.
- Outerra forum >
- Anteworld - Outerra Game >
- Modding: Importer, Tools & Utilities >
- JavaScript development