Outerra forum
- July 01, 2025, 09:01:47 am
- Welcome, Guest
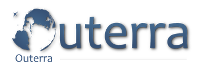
News:
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.
- Outerra forum >
- Anteworld - Outerra Game >
- Modding: Importer, Tools & Utilities >
- JavaScript development