Outerra forum
- April 05, 2025, 07:49:55 am
- Welcome, Guest
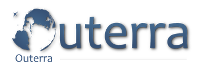
News:
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.
- Outerra forum >
- Outerra Engine >
- Development screen shots and videos >
- Importer and vehicle physics video
Author
Topic: Importer and vehicle physics video (Read 78840 times)
- Outerra forum >
- Outerra Engine >
- Development screen shots and videos >
- Importer and vehicle physics video