Outerra forum
- July 11, 2025, 07:41:18 am
- Welcome, Guest
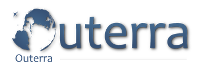
News:
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.