Outerra forum
- May 24, 2025, 03:44:35 am
- Welcome, Guest
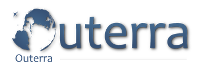
News:
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.