Outerra forum
- July 16, 2025, 12:15:11 am
- Welcome, Guest
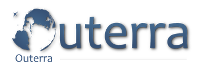
News:
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.
Download Outerra Tech Demo. Unofficial Outerra Discord server, MicroProse Discord server for OWS.